I Can Has Cheezburger?
A graphical interface to get some fast food
Sébastien Boisgérault
Associate Professor, ITN Mines Paris – PSL
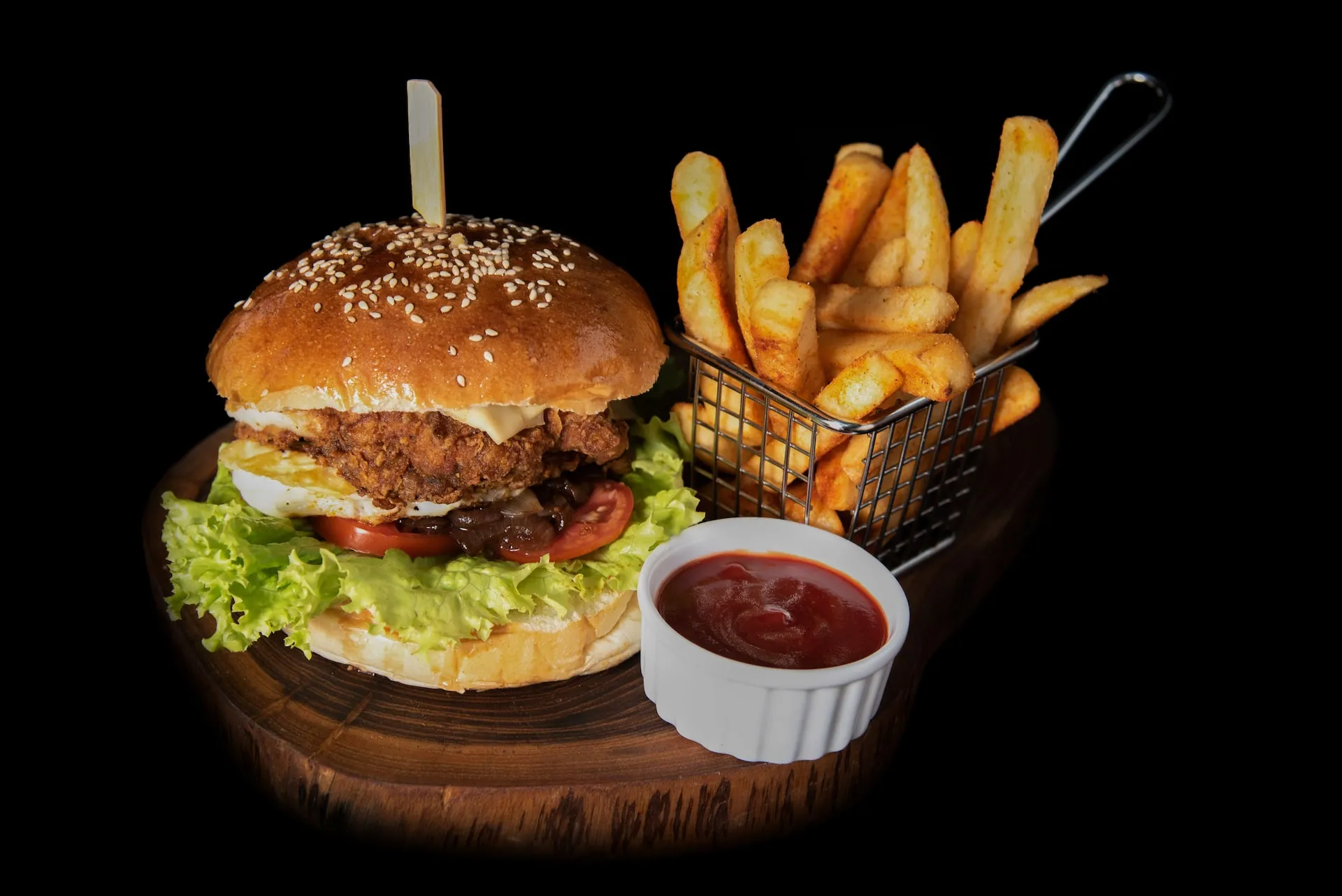
At the beginning …
Flet is a Python library for building graphical user interfaces.
Implement the example given in its documentation to get familiar with it.
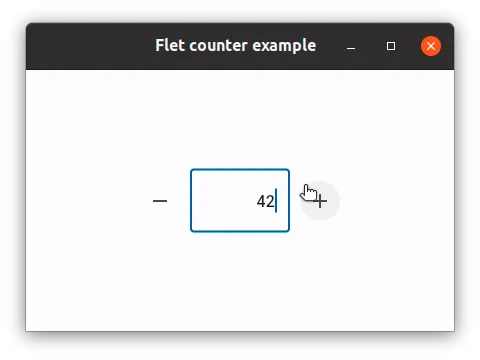
Graphical Architecture
Develop the graphical architecture of a menu ordering application, represented in the figure below:
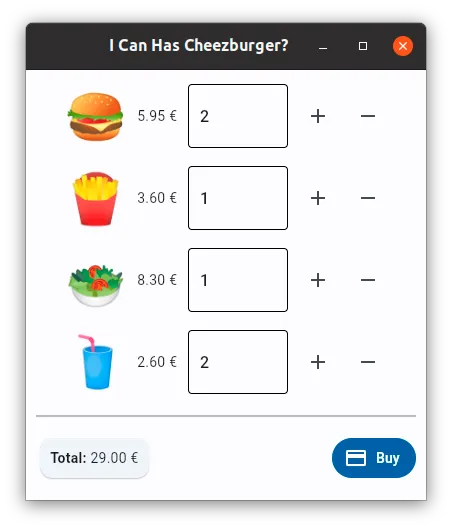
You will have to explore the documentation of the components offered by flet.
At first:
-
Nothing is “functional” in your interface: all numeric values are coded “hard-coded”, nothing happens when you click on a button, etc.
-
Don’t worry if the appearance of your interface is not exactly the same as the one given in the example. It will always be time to come back later to refine this representation.
You can use https://emojipedia.org to find the emojis you need (🍔 hamburger, 🍟 fries, etc.)
Custom Component
The flet documentation explains how you can create your own components. Used wisely, this possibility should allow you to make the architecture of your command application more readable.
Ideally, we would like to have a Product
component that takes care of
the representation of a product, the display of its price as well as the counting
of the number of units that the customer wishes to order. The resulting application
could then take the following form:
from flet import app, icons
from flet import MainAxisAlignment
from flet import (
Card,
Column,
Container,
Divider,
FilledButton,
IconButton,
Markdown,
Row,
Text,
TextField,
)
from product import Product
def main(page):
page.title = "I Can Has Cheezburger?"
page.window_width = 400
page.window_height = 430
page.add(
Column(
alignment=MainAxisAlignment.CENTER,
controls=[
Product("🍔", 5.95),
Product("🍟", 3.60),
Product("🥗", 8.30),
Product("🥤", 2.60),
Divider(),
Row(
[
Card(
Container(
Markdown(
"**TOTAL:** 0.00 €"
),
padding=10,
)
),
FilledButton(
text="Buy", icon=icons.PAYMENT
),
],
alignment=MainAxisAlignment.SPACE_BETWEEN,
),
],
)
)
app(target=main)
Develop a class Product
in a file product.py
so that this new program works
(as before).
Locally Functional Component
Make sure that the Product
component is functional locally. That is to say,
that the buttons +
and -
of a product only affect the quantity of this product
and not the quantity of another product. Do not worry about the total of the order
for the moment. However, make sure that the quantity of a product cannot be negative.
Fully Functional Component
Two things are missing from our product component:
-
An attribute (or property)
total
that allows to know how much the chosen number of units of this component will cost.hamburgers = Product("🍔", 5.95) hamburgers.total # 0.0 initially
-
A (optional) hook to signal to the user of the component that the number of units (and therefore the cost) of this product has changed. This hook will take the form of a callback function that we provide to the product when it is constructed:
def print_hamburgers_total(event): print(hamburgers.total) hamburgers = Product("🍔", 5.95, on_change=print_hamburgers_total)
Make the necessary changes to the Product
component so that it is fully functional.
Integration
Complete your application so that the total of the order is always up to date.