Lost
When your snake wanders in a maze...
Sébastien Boisgérault
Associate Professor, ITN Mines Paris – PSL
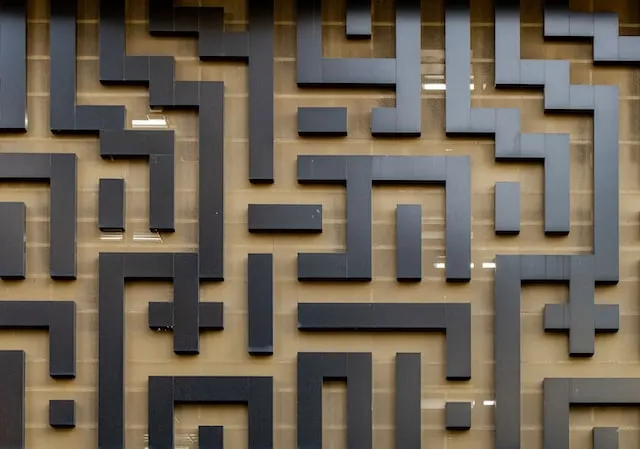
Introduction
The aim of this project is to develop an “automatic navigation system” for our snake, to allow it to reach the fruit despite a complex environment with rocks that should be avoided.
Getting Started
For the sake of simplicity, we will consider here only mazes defined within
a 30x30 grid. We will represent them in Python as sets of pairs of integers
between 0 and 29, where each pair represents the coordinates of an empty
cell of the maze.
The pair (0, 0)
corresponds to the top left corner of the grid.
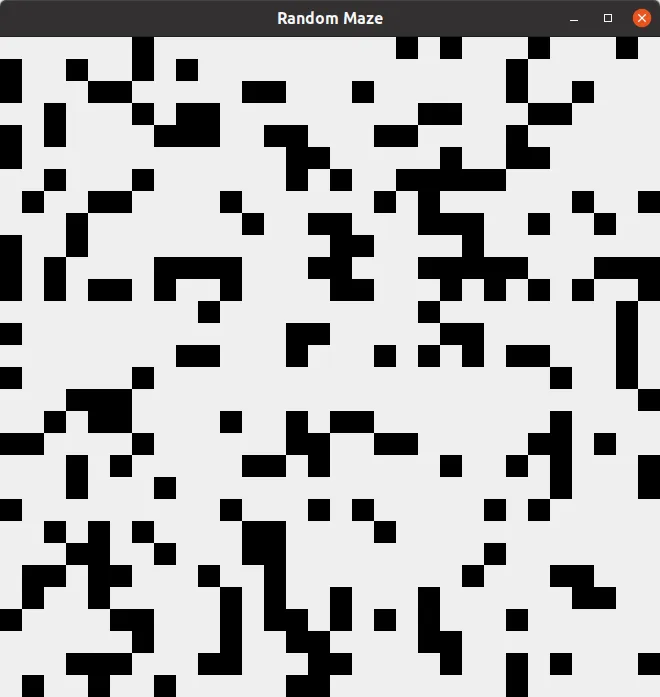
A maze generated (pseudo-)randomly with a mix of 75% empty cells (in white) and 25% walls (in black).
Maze Visualizer
We store the description of such mazes in files, using a
simple (but undocumented) file format.
For example the maze displayed above is stored as random-maze.py
.
-
Download this example maze file.
-
Inspect it contents and make an educated guess about the file format specification.
-
Construct the maze object
random_maze
associated to this file.
Visualization
Develop a function draw_maze
to display a maze.
This function should work in the following context:
import pyxel
WIDTH = 30
HEIGHT = 30
random_maze = ...
pyxel.init(WIDTH, HEIGHT)
draw_maze(random_maze)
pyxel.show()
Create your own maze
Design your own 30x30 maze, for example the empty maze, a spiral, etc.
Graphs and Paths
Mazes and Graphs
For each maze we define a graph
-
whose vertices are the empty cells of the maze,
-
whose edges represent the admissible movements from one cell to a neighboring cell (the two cells are empty and share a side),
-
for which the weight of each edge is 1; it represents the number of elementary actions (or “cost”) needed to move from one cell to a neighboring cell.
Given this definition
-
What Python data structure would we naturally use to represent these graphs?
-
Implement a function
maze_to_graph
which builds the graph associated to a maze.
Reachable Cells
Implement a function reachable_cells
which returns the set
of cells of a maze which are reachable from
the cell source
.
Computation of the cells reachable from the top-left corner. The green cells are reachable cells whose neighbors have between inspected; orange cells are reachable, but their neighbors have not yet been inspected; Two groups of empty cells stay white until the end, and hence are unreachable.
Extend the function draw_maze
to graphically differentiate
a set of cells using a given color.
Then use it to represent the set
of cells reachable from the top left corner of the maze
random_maze
.
Mazes and Paths
Computation of the cells reachable from the top-left corner, together with the path effectively used to joing them (in pink).
Use this function to find a path joining the top left and bottom right corners
of the maze random_maze
and graphically represent the result by updating
your function draw_maze
.
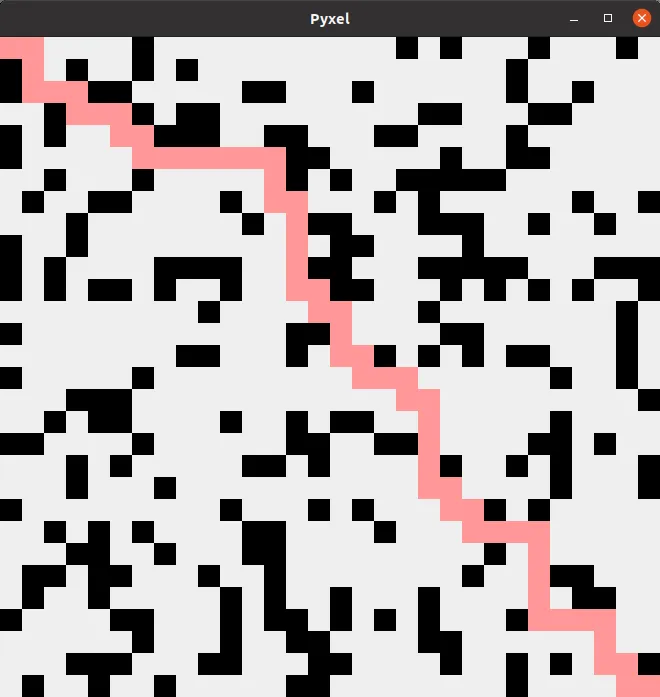
A path that joins the top left corner and the bottom right one (in pink).
Snake Game: Autopilot
Go back to the snake game of the previous session, discard the user inputs and instead use your brand new path finding algorithm to select the snake next move.